memo Nouveau venu


Nombre de messages : 18 Age : 37 Localisation : devant mon pc Date d'inscription : 02/02/2010
![[RMXP]Map Zoom Empty](https://2img.net/i/empty.gif) | Sujet: [RMXP]Map Zoom Jeu 11 Fév - 0:57 | |
| Map ZoomVersion: 1.1 ![[RMXP]Map Zoom Scriptzoom1ji0](https://2img.net/r/ihimizer/img110/4342/scriptzoom1ji0.png) IntroductionVous permez de zoomer sur un point précis de la map, avec une vitesse variable. Démo Lien Attention c'est en anglais. Script Vous devez posséder le script "SDK " version 2.2, et ce script Tilemap v1.0 SDK La démo contient tout ce qu'il faut Code: - Spoiler:
=begin ============================================================================== ** Map_Zoom ------------------------------------------------------------------------------ Trebor777 Version 1.1 14/06/2007 ------------------------------------------------------------------------------ * Description FR:
Ce script permet d'effectuer des zoom in et zoom out sur un point pr?cis de la map.
* Description US:
This script allow you to zoom in or out on a specific map location.
------------------------------------------------------------------------------ * Instructions FR:
Placer le Script sous le SDK et au dessus de Main. Utiliser 1 des 2 commandes dans un script ou un ?v?nement "appeler un script": . $game_map.start_zoom(x,y,coef,spd) . $scene.zoom(x,y,coef,spd) #avec $scene une instance de Scene_Map
x et y: Coordonn?es(pas en pixel) du point ? zoomer/d?zoomer coef : Valeur du zoom ? atteindre de 0.1, ? l'infini spd : Vitesse du zoom, la variation est bas?e sur le calcul suivant: 0.2*spd
* Instructions US:
Place The Script Below the SDK and Above Main. Use 1 of this 2 command in a script or with the event command "Call a script": . $game_map.start_zoom(x,y,coef,spd) . $scene.zoom(x,y,coef,spd) #with $scene a Scene_Map instance
x & y: Target Location(not inpixel)to zoom in/out coef : Zoom value to reach from 0.1, to infinite spd : Zoom speed, the fluctuation is based on this formula: 0.2*spd Version 1.1 Code adapted to the new tilemap class. ============================================================================== =end #------------------------------------------------------------------------------ # * SDK Log Script #------------------------------------------------------------------------------ SDK.log('Map_Zoom', 'Trebor777', 1.1, '14-06-2007')
#------------------------------------------------------------------------------ # * Begin SDK Enable Test #------------------------------------------------------------------------------ if SDK.state('Map_Zoom') and SDK.state('Tilemap')
#============================================================================== # ** Spriteset_Map #============================================================================== class Spriteset_Map def update_character_sprites # Update character sprites for sprite in @character_sprites sprite.zoom_x=$game_map.tilemap_settings.zoom_x sprite.zoom_y=$game_map.tilemap_settings.zoom_y sprite.update end end end #============================================================================== # ** Scene_Map #============================================================================== class Scene_Map def zoom(x,y,coef,spd) if !$game_map.zooming? $game_map.start_zoom(x,y,coef,spd) end end end #============================================================================== # ** Game_Map #============================================================================== class Game_Map #-------------------------------------------------------------------------- alias zoom_setup_scroll setup_scroll def setup_scroll setup_zoom zoom_setup_scroll end #-------------------------------------------------------------------------- def setup_zoom # Initialize scroll information @zoom_rest = 0 @zoom_speed = 2 end #-------------------------------------------------------------------------- # * Start Zoom # focus : zoom value to reach # speed : zoom speed #-------------------------------------------------------------------------- def start_zoom(x,y,focus, speed) @x_zoom=x @y_zoom=y @zoom_rest = (focus-@tilemap_settings.zoom_x).abs @zoom_speed = speed*((focus-@tilemap_settings.zoom_x)/@zoom_rest) end #-------------------------------------------------------------------------- # * Determine if Scrolling #-------------------------------------------------------------------------- def zooming? return @zoom_rest > 0 end #-------------------------------------------------------------------------- alias zoom_update_scrolling update_scrolling def update_scrolling update_zooming zoom_update_scrolling end #-------------------------------------------------------------------------- def update_zooming # If zooming if @zoom_rest > 0 # Change from zoom speed to focus in map coordinates focus = 0.2 * @zoom_speed # Execute zooming @tilemap_settings.zoom_x =focus @tilemap_settings.zoom_y =focus # Subtract focus zoomed @zoom_rest -= focus.abs $game_player.center(@x_zoom,@y_zoom) end end #-------------------------------------------------------------------------- # * Scroll Down # distance : scroll distance #-------------------------------------------------------------------------- def scroll_down(distance) @display_y = [@display_y distance, (self.height*@tilemap_settings.zoom_y - 15) * 128/tilemap_settings.zoom_y].min end #-------------------------------------------------------------------------- # * Scroll Left # distance : scroll distance #-------------------------------------------------------------------------- def scroll_left(distance) @display_x = [@display_x - distance, 0].max end #-------------------------------------------------------------------------- # * Scroll Right # distance : scroll distance #-------------------------------------------------------------------------- def scroll_right(distance) @display_x = [@display_x distance, (self.width*@tilemap_settings.zoom_x - 20) * 128/tilemap_settings.zoom_x].min end #-------------------------------------------------------------------------- # * Scroll Up # distance : scroll distance #-------------------------------------------------------------------------- def scroll_up(distance) @display_y = [@display_y - distance, 0].max end end #============================================================================== # ** Game_Character #============================================================================== class Game_Character #-------------------------------------------------------------------------- # * Get Screen X-Coordinates #-------------------------------------------------------------------------- def screen_x # Get screen coordinates from real coordinates and map display position #return (@real_x) / 4 # 16 return (@real_x - $game_map.display_x 3*$game_map.tilemap_settings.zoom_x) / 4*$game_map.tilemap_settings.zoom_x 16*$game_map.tilemap_settings.zoom_x end #-------------------------------------------------------------------------- # * Get Screen Y-Coordinates #-------------------------------------------------------------------------- def screen_y # Get screen coordinates from real coordinates and map display position y = (@real_y - $game_map.display_y 3*$game_map.tilemap_settings.zoom_y) / 4*$game_map.tilemap_settings.zoom_y 32*$game_map.tilemap_settings.zoom_y # Make y-coordinate smaller via jump count if @jump_count >= @jump_peak n = @jump_count - @jump_peak else n = @jump_peak - @jump_count end return y - (@jump_peak * @jump_peak - n * n) / 2 end #-------------------------------------------------------------------------- # * Get Screen Z-Coordinates #-------------------------------------------------------------------------- def screen_z(height = 0) # If display flag on closest surface is ON if @always_on_top # 999, unconditional return 999 end # Get screen coordinates from real coordinates and map display position z = (@real_y - $game_map.display_y 3*$game_map.tilemap_settings.zoom_y) / 4*$game_map.tilemap_settings.zoom_y 32*$game_map.tilemap_settings.zoom_y # If tile if @tile_id > 0 # Add tile priority * 32 return z $game_map.priorities[@tile_id] * 32*$game_map.tilemap_settings.zoom_y # If character else # If height exceeds 32, then add 31 return z ((height > 32) ? 31 : 0) end end end #============================================================================== # ** Game_Player #============================================================================== class Game_Player < Game_Character @center_x= CENTER_X # Center screen x-coordinate * 4 @center_y = CENTER_Y # Center screen y-coordinate * 4 #-------------------------------------------------------------------------- # * Scroll Down #-------------------------------------------------------------------------- def update_scroll_down(last_real_y) # If character moves down and is positioned lower than the center # of the screen if @real_y > last_real_y and @real_y - $game_map.display_y > @center_y # Scroll map down $game_map.scroll_down(@real_y - last_real_y) end end #-------------------------------------------------------------------------- # * Scroll Left #-------------------------------------------------------------------------- def update_scroll_left(last_real_x) # If character moves left and is positioned more let on-screen than # center if @real_x < last_real_x and @real_x - $game_map.display_x < @center_x # Scroll map left $game_map.scroll_left(last_real_x - @real_x) end end #-------------------------------------------------------------------------- # * Scroll Right #-------------------------------------------------------------------------- def update_scroll_right(last_real_x) # If character moves right and is positioned more right on-screen than # center if @real_x > last_real_x and @real_x - $game_map.display_x > @center_x # Scroll map right $game_map.scroll_right(@real_x - last_real_x) end end #-------------------------------------------------------------------------- # * Scroll Up #-------------------------------------------------------------------------- def update_scroll_up(last_real_y) # If character moves up and is positioned higher than the center # of the screen if @real_y < last_real_y and @real_y - $game_map.display_y < @center_y # Scroll map up $game_map.scroll_up(last_real_y - @real_y) end end #-------------------------------------------------------------------------- # * Set Map Display Position to Center of Screen #-------------------------------------------------------------------------- def center(x, y) @center_x= (320/$game_map.tilemap_settings.zoom_x - 16) * 4 # Center screen x-coordinate * 4 @center_y = (240/$game_map.tilemap_settings.zoom_y - 16) * 4 # Center screen y-coordinate * 4 max_x = ($game_map.width*$game_map.tilemap_settings.zoom_x - 20) * 128/$game_map.tilemap_settings.zoom_x max_y = ($game_map.height*$game_map.tilemap_settings.zoom_y - 15) * 128/$game_map.tilemap_settings.zoom_y $game_map.display_x = ([0, [x * 128 - @center_x, max_x].min].max) $game_map.display_y = ([0, [y * 128 - @center_y, max_y].min].max) end end #-------------------------------------------------------------------------- # * End SDK Enable Test #-------------------------------------------------------------------------- end
InstructionsLisez l'entête en commentaires^^ Et dans le script Tilemap, vous devez le réactiver. Sinon mon script ne fonctionnera pas: recherchez la ligne (en début du script): "SDK.disable('Tilemap')" Et commentez cette ligne en ajoutant un # devant celle-ci. | |
|
Naqqah Administrateur
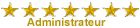

Nombre de messages : 844 Age : 28 Localisation : Parti manger un ours Date d'inscription : 22/02/2009
![[RMXP]Map Zoom Empty](https://2img.net/i/empty.gif) | Sujet: Re: [RMXP]Map Zoom Jeu 11 Fév - 20:30 | |
| Merci beaucoup pour ce partage.
Perso, il me servira mais ça peut être utile pour des jeux style infiltration ou point & click. | |
|
kilam1110 Webmaster
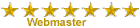

Nombre de messages : 1165 Age : 104 Localisation : Devant mon ordi Date d'inscription : 12/02/2009
![[RMXP]Map Zoom Empty](https://2img.net/i/empty.gif) | Sujet: Re: [RMXP]Map Zoom Ven 12 Fév - 18:37 | |
| Merci de remplir cette section memo  | |
|
Naqqah Administrateur
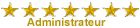

Nombre de messages : 844 Age : 28 Localisation : Parti manger un ours Date d'inscription : 22/02/2009
![[RMXP]Map Zoom Empty](https://2img.net/i/empty.gif) | Sujet: Re: [RMXP]Map Zoom Ven 12 Fév - 20:20 | |
| Ah oui j'y pense, vu que je vais pas faire les modif's à tous les sujets : mettre le nom du log pour lequel est fait le script !
Vexkar, si tu peux faire un note quelque part pour que tout le monde le sache... | |
|
Timo8562 Nouveau venu

Nombre de messages : 6 Age : 27 Localisation : Chez moi Date d'inscription : 10/02/2011
![[RMXP]Map Zoom Empty](https://2img.net/i/empty.gif) | Sujet: Re: [RMXP]Map Zoom Mer 22 Juin - 20:12 | |
| Le lien de la démo est mort ? | |
|
kilam1110 Webmaster
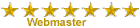

Nombre de messages : 1165 Age : 104 Localisation : Devant mon ordi Date d'inscription : 12/02/2009
![[RMXP]Map Zoom Empty](https://2img.net/i/empty.gif) | Sujet: Re: [RMXP]Map Zoom Mer 22 Juin - 20:15 | |
| | |
|
Timo8562 Nouveau venu

Nombre de messages : 6 Age : 27 Localisation : Chez moi Date d'inscription : 10/02/2011
![[RMXP]Map Zoom Empty](https://2img.net/i/empty.gif) | Sujet: Re: [RMXP]Map Zoom Mer 22 Juin - 20:25 | |
| F****, j'espérais enfin avoir trouver ce que je cherche. Je vais essayer de retrouver la source. | |
|
kilam1110 Webmaster
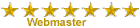

Nombre de messages : 1165 Age : 104 Localisation : Devant mon ordi Date d'inscription : 12/02/2009
![[RMXP]Map Zoom Empty](https://2img.net/i/empty.gif) | Sujet: Re: [RMXP]Map Zoom Mer 22 Juin - 20:53 | |
| Bah le script est fait pour être copié sur un nouveau projet, non ? | |
|
Blazeon Nouveau venu

Nombre de messages : 1 Age : 2015 Localisation : Russia Date d'inscription : 18/12/2013
![[RMXP]Map Zoom Empty](https://2img.net/i/empty.gif) | Sujet: Re: [RMXP]Map Zoom Mer 18 Déc - 21:28 | |
| Hello, sorry, have to speak english, i'm from Russia. A have a question about your 'map zoom' script. When I used it in a project and launched test game, it has shut and gave me "script ' ' line 137: SyntaxError occurred." error. What can you advice me to make this script work? I think it could've been that I have to use SDK script. Is it possible to use Map Zoom without SDK or make a new version? Please help, i really need it! | |
|
Contenu sponsorisé
![[RMXP]Map Zoom Empty](https://2img.net/i/empty.gif) | Sujet: Re: [RMXP]Map Zoom ![[RMXP]Map Zoom Empty](https://2img.net/i/empty.gif) | |
| |
|